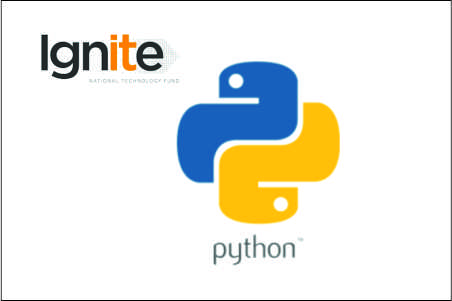
TO BE SCHEDULED AS YET
This course contains a basic level content for Arabic language & has been organized with reference to the Quranic verses used in prayers (Namaz).
Embarking on a journey to master the intricacies of supply chain and operations management offers an unparalleled opportunity to delve into the theoretical underpinnings and strategic frameworks that govern global commerce. This course is meticulously designed to equip future leaders with a profound understanding of the interconnected networks and processes that drive the production and distribution of goods and services. Through a comprehensive curriculum, students will explore the critical concepts and methodologies that form the backbone of efficient and effective supply chain management. What you will learn Understand fundamental supply chain management theories and concepts.Explore classical and contemporary supply chain models.Analyze the structure and optimization of supply networks.Examine strategic elements of operations management.Learn decision-making processes for successful operations strategies.Gain insights into resource allocation and process innovation.Study case studies to anticipate challenges in operations.Delve into logistics and transportation theories in supply chains.Understand factors influencing global logistics and transportation.Explore inventory management theories for supply chain efficiency.Learn to balance supply and demand in inventory strategies.Investigate supply chain integration and collaboration theories.Promote cohesive efforts among supply chain partners.Foster analytical and strategic thinking for leadership roles.Develop a robust theoretical framework for global supply chains.Acquire intellectual tools for success in supply chain management. Skills you'll gain Strategic Supply Chain PlanningOperations and Process OptimizationInventory & Warehouse ManagementLogistics and Distribution StrategyProcurement and Supplier Relationship ManagementDemand Forecasting and Capacity PlanningLean Management and Six Sigma TechniquesRisk Management in Supply ChainsERP Systems and Supply Chain TechnologiesData-Driven Decision Making using AnalyticsGlobal Supply Chain IntegrationProject and Change ManagementQuality Control and Continuous ImprovementSustainable and Ethical Supply Chain PracticesLeadership & Communication in Operations Environments Pre-requisite No prior experience in supply chain or operations management is required—just an interest in how goods and services move efficiently through global networks.A basic understanding of business or logistics concepts can be helpful, but the course starts with fundamental theories to ensure accessibility for all learners.Participants should have analytical thinking skills and a curiosity about optimizing processes and decision-making in supply chain management.A willingness to engage with case studies and theoretical models will enhance understanding of real-world supply chain challenges and solutions.Enthusiasm for learning about logistics, inventory management, and operational strategies will be beneficial in applying course concepts to practical scenarios. Certificate Add this credential to your LinkedIn profile, resume, or CV. Share it on social media and in your performance review. It is a proof for an employer, school, or other institution that you have successfully completed an online course. Career Paths after Completing This Course Supply Chain Manager – Oversee end-to-end supply chain operationsOperations Manager – Improve processes, productivity, and logisticsProcurement Manager – Manage sourcing, purchasing, and vendor relationshipsLogistics Manager – Coordinate transportation, warehousing, and distributionInventory Control Manager – Optimize stock levels and warehouse efficiencyProduction Planner – Ensure timely manufacturing and resource allocationSupply Chain Analyst – Use data to improve supply chain performanceConsultant / Analyst – Advise companies on streamlining operations Perfect for careers in manufacturing, retail, e-commerce, logistics, healthcare, and multinational companies. This qualification is highly valued for leadership roles in operational and global supply chain strategies.
This course is designed to equip learners with essential communication and presentation skills tailored for the corporate world. Whether you're entering the job market or aiming to enhance your workplace communication, this 18-week bilingual course (Urdu/English) blends theory with practical applications, offering real-world scenarios, interactive sessions, and assignments that will elevate your confidence and performance in professional settings. You'll learn how to write professionally, speak persuasively, handle corporate conversations, and deliver compelling presentations that leave a lasting impression. What you will learn Foundations of effective corporate communicationVerbal and non-verbal communication techniquesEmail and business writing etiquettePresentation design and delivery skillsHandling professional meetings and negotiationsConflict resolution and feedback deliveryBuilding confidence in public speakingCross-cultural workplace communication Skills you'll gain Communication confidenceBusiness writing proficiencyPublic speaking and presentation masteryNegotiation and persuasionTeam collaboration and leadership communicationTime-bound professional expression Pre-requisite No prior experience required. Just a willingness to learn and participate. Certificate Add this credential to your LinkedIn profile, resume, or CV. Share it on social media and in your performance review. It is a proof for an employer, school, or other institution that you have successfully completed an online course. Career Paths after Completing This Course Upon completing this course, learners will: Deliver professional, persuasive, and engaging presentations in real-world business settingsStructure ideas clearly and communicate with confidence, clarity, and charismaUse body language, voice modulation, and visuals to influence and inspire audiencesHandle feedback, questions, and challenges during presentations with professional poiseStand out in meetings, pitches, interviews, and client interactionsGain a Certificate of Completion from Iqrasity.org to showcase workplace communication expertise This course empowers participants to become dynamic presenters and effective communicators—essential skills for leadership roles, corporate success, and career advancement.
This all-in-one IELTS preparation course is designed to help students excel in Listening, Reading, Writing, and Speaking. With a balance of live sessions, recorded lectures, practical manuals, assignments, and quizzes, learners receive personalized support and weekly challenges. The course includes 16 weekly modules, a midterm, and a final exam, preparing students thoroughly for either the Academic or General IELTS exams. What you will learn Complete understanding of IELTS exam structure and scoringStrategies to solve each question type across all sectionsTechniques to improve listening comprehension and note-takingAdvanced reading skills like skimming, scanning, and inferenceAcademic and general writing mastery (Task 1 & Task 2)Confidence and fluency in speaking with band-descriptor alignmentTime management and exam practice through full mocks Skills you'll gain Test-taking strategies for IELTSAcademic vocabulary and grammarCritical reading and analysisStructured writing for tasks 1 and 2Fluent spoken English with better pronunciationSelf-assessment and performance tracking Pre-requisite Basic knowledge of English Certificate Add this credential to your LinkedIn profile, resume, or CV. Share it on social media and in your performance review. It is a proof for an employer, school, or other institution that you have successfully completed an online course. Career Paths after Completing This Course After successfully completing of this course, learners will: Be fully equipped to achieve a Band 7.5–8+ in all four IELTS modules (Listening, Reading, Writing & Speaking)Communicate confidently in academic, professional, and immigration settingsMaster advanced English skills—grammar, vocabulary, coherence, fluency, and pronunciationApproach the IELTS test with a winning strategy, time management skills, and mock test experienceBe prepared for global opportunities—including international university admissions, work visas, scholarships, and immigration pathwaysReceive a Certificate of Completion from Iqrasity.org to showcase language proficiency and commitment to growth Whether you aim to study abroad, advance your career, or relocate internationally, this course becomes your passport to global success.