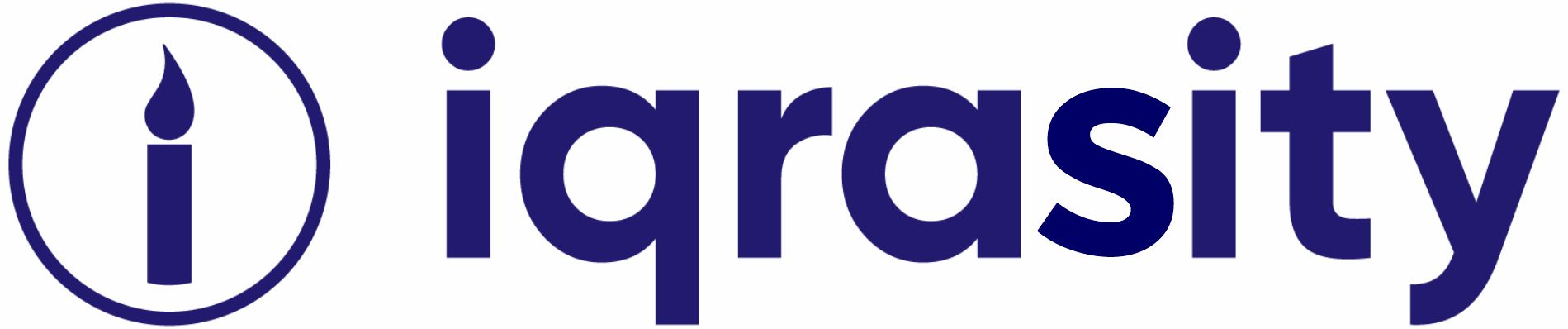
- History and evolution of Java
- Features and advantages
- Basic syntax and data types (primitive types, operators)
- Variables, control structures (if/else, switch, loops)
- Functions (methods) and modules (classes)
- Classes and objects (attributes, methods, constructors)
- Inheritance (single and multiple inheritance)
- Polymorphism (method overriding, method overloading)
- Encapsulation and abstraction
- Abstract classes and interfaces
- Primitive types (int, double, char, boolean)
- Reference types (String, Arrays, ArrayList)
- Operators (arithmetic, comparison, logical, assignment)
- Control structures (if/else, switch, loops)
- Method declarations and calls
- Method overloading and overriding
- Functional programming (lambda expressions, method references)
- Exception handling (try-catch, throw)
- Arrays and ArrayList
- LinkedList and HashSet
- TreeSet and TreeMap
- Collection interfaces (Iterable, Collection, List)
- Reading and writing text files (BufferedReader, BufferedWriter)
- Reading and writing object files (Serialization)
- Database connectivity (JDBC)
- File input/output best practices
- Thread creation and management
- Synchronization (locks, semaphores)
- Deadlocks and starvation
- Concurrent collections and interfaces
- Generics and wildcards
- Annotations and reflection
- Lambda expressions and functional programming
- Java 8 features (Stream API, functional programming)
- Introduction to Servlet and JSP
- Building web applications (MVC pattern)
- Web development best practices
- Building a full-stack project (console, web, or mobile)
- Project planning and management
- Debugging and testing
- Error handling and debugging
- Testing and automation (JUnit, TestNG)
- Best practices and coding standards
- Advanced libraries and frameworks (Spring, Hibernate)